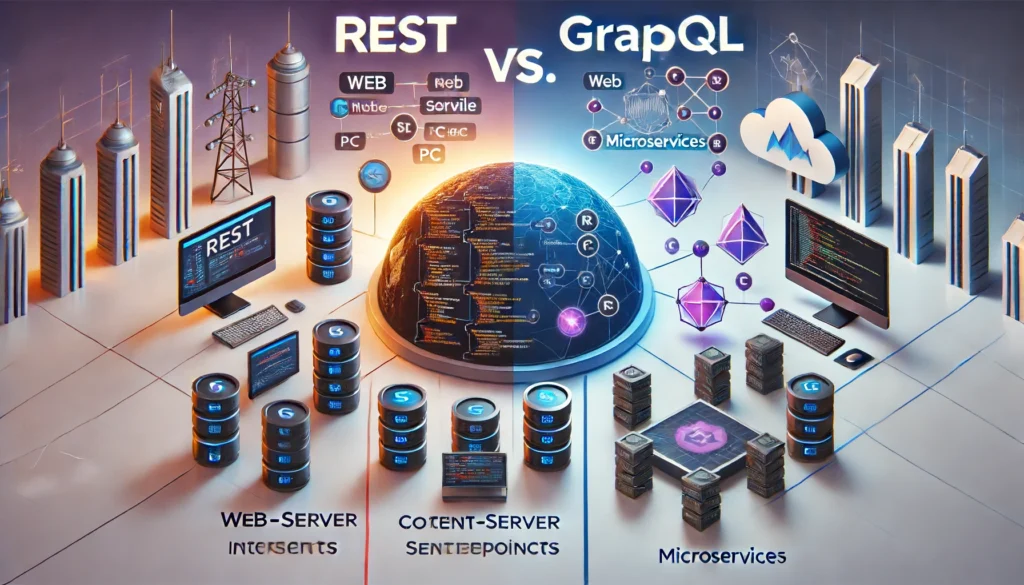
In the world of APIs, two dominant approaches stand out: REST and GraphQL. Each has its unique advantages and use cases, and understanding their differences can help you choose the right tool for your project. Let’s delve into a comparative analysis based on the provided image.
Understanding REST
REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on a stateless, client-server communication protocol, typically HTTP. Here’s how it works:
- Client Request: The client (which could be a web browser, mobile app, or Desktop PC) sends a request to retrieve data. For example, a request to get user information:
GET /users/123
. - Server Response: The server responds with the requested data. For instance, the user information is returned in JSON format:
{
"name": "Bob",
"gender": "male",
"GET /orders/456"
}
- Additional Requests: If more data is needed (e.g., the user’s orders), the client makes additional requests:
GET /orders/456
. - Further Response: The server provides the additional requested data, again in JSON format:
{
"product": "abc",
"quantity": 2,
"price": "100.00"
}
This process illustrates the typical multiple request-response cycles required in REST to gather related data from various endpoints.
Exploring GraphQL
GraphQL is a query language for APIs and a runtime for executing those queries. It allows clients to request exactly the data they need, potentially from multiple sources, in a single request. Here’s how it functions:
- Client Request: The client sends a single request to the GraphQL endpoint. For example:
{
user(id: 123) {
name
gender
order {
product
quantity
price
}
}
}
- GraphQL Server: The GraphQL server processes the query by interacting with various services or databases.
- Data Aggregation: The server fetches the required data, often from multiple microservices such as User Service and Order Service.
- Single Response: The server sends back a consolidated response containing all the requested data:
{
"user": {
"id": "123",
"name": "Bob",
"gender": "male",
"order": {
"product": "abc",
"quantity": 2,
"price": "100.00"
}
}
}
This approach minimizes the number of requests and provides a more efficient way to fetch related data.
Key Differences and Considerations
- Number of Requests:
- REST: Often requires multiple requests to different endpoints to fetch related data.
- GraphQL: Typically requires a single request to fetch all related data.
- Data Fetching:
- REST: Retrieves fixed data structures from specific endpoints.
- GraphQL: Allows clients to specify exactly what data they need, reducing over-fetching and under-fetching of data.
- Flexibility:
- REST: Adding new endpoints or modifying existing ones can be cumbersome as it often requires changes on both client and server sides.
- GraphQL: Offers greater flexibility in evolving APIs without impacting existing queries.
- Complexity:
- REST: Simpler to implement and understand for basic use cases.
- GraphQL: Requires more setup and learning but provides powerful querying capabilities.
Conclusion
Choosing between REST and GraphQL depends on your project requirements. REST is straightforward and effective for simple, well-defined interactions. GraphQL, on the other hand, offers more flexibility and efficiency for complex data fetching needs, especially when dealing with interconnected resources.
Understanding these differences will help you make an informed decision about which approach to use in your next project. Whether you prefer the simplicity of REST or the power of GraphQL, both have their place in modern API design.